Ladder / Railings
A ladder is a game object on which character can climb up and down.
Player is snapped onto ladder as soon as it lies completely into ladder box collider zone and if pushing vertical axis (unless autocatch is enabled).
You must adds a BoxCollider2D and attach the ladder scripts to enable ladder.
BoxCollider must be flagged as "Is Trigger" or be put into non colliding physic layer to prevent character from colliding with it.
Same rules apply to railings except that player can move freely on horizontal axis too.
More over you can add many BoxColliders to handle complex railings (see Basics demo level).
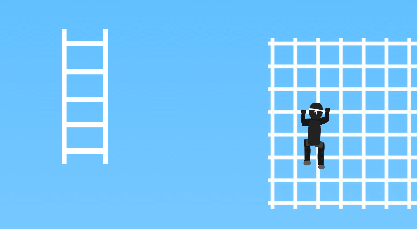
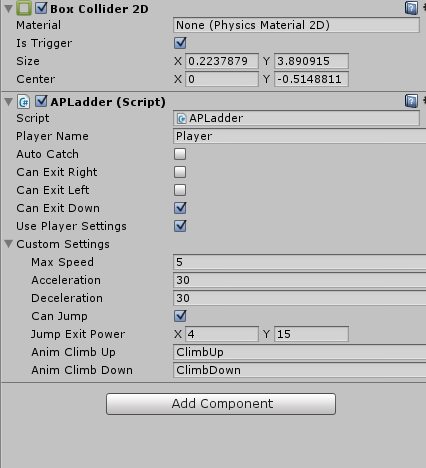
-
Player Name : name of player to survey to catching
-
Auto Catch : player is catched as soon as overlapping box collider zone, otherwise he must push up axis
-
Can Exit XXX : allows player to exit in XXX direction if trying to
-
Use Player Settings : use default player settings or Custom Settings defined below
Default settings regarding behavior on a ladder/railings are carried by the CharacterController script.
But each one can be overridden by game object if needed.
For example we could make a special ladder on which we move slower than others.
You just have to uncheck "Use Player Settings" on concerned ladder/railings and tweak custom settings for it.

-
Max Speed : maximum speed (m/s)
-
Acceleration : acceleration (m/s²)
-
Deceleration : deceleration (m/s²)
-
Can Jump : allows jumping in facing direction while catched
-
Jump Exit Power : jump power
-
Anim Climb XXX : animation to use for moving in XXX direction
Carriers
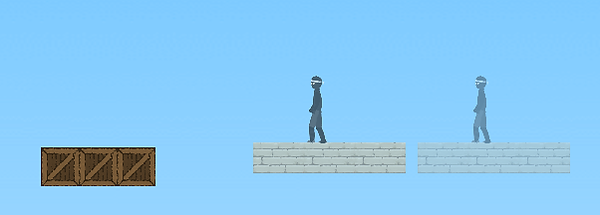
When lying on a moving object, your character may not be carried.
To enable this, you have to attach a APCarrier script on it.
Then you have 2 options :
-
your carrier object is moved by native Unity animation system:
-
make sure that Animate Physics is checked in the Animation properties
-
check the box Animation Mode on the APCarrier script
-
-
carrier object is updated by code in a FixedUpdate method (like in the SampleMovingObject script):
-
uncheck Animation Mode on the APCarrier script.
-
make sure that your script is executed before APCarrier script (Edit > Project Settings > Script Execution Order), by default this should be ok
-
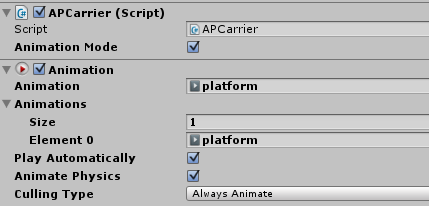
Camera
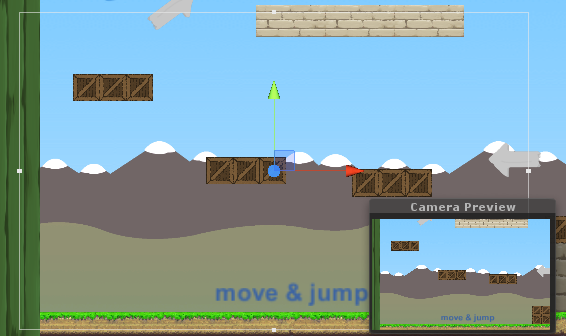
Provided camera should fits most need of any 2D platformer.
This fits standard 'Mario' style camera. Please have a look here for concise explanation : the-ideal-platformer-camera-should-minimize
Attach it to your game camera and select target transform to use.

-
Margin X/Y : allowed inside margin for Player in which camera does not move
-
Offset : add static offset from Player
-
Face Lead : camera horizontal offset when Player is moving forward
-
Face Lead Power : power used to apply Face Lead offset
-
Speed : camera speed in function of distance to Player
-
Clamp Enabled : enable camera position clamping
-
Min Pos / Max Pos : min/max camera position when clamp is enabled
-
Clamp Update Speed : applied only when new clamp values are specified at runtime, used to smooth transition to new values
-
Player : transform to follow
-
Parallax Scrolling : enable parallax scrolling on specified transform using this camera
-
Ratio : speed ratio of horizontal scrolling, must be in [0,1] range. 1 means we follow camera at its full speed, 0 means we never move
-
Ratio Vertical : same as above but for vertical scrolling
-
Target : target transform to update
-
Jumper
This special game object (which could have been a sample game object) behaves like a jump effector when character touches it.
An animation is played once when touched by the character.
You can choose the direction and power of impulse if you are in an Impulse Mode.
Otherwise this is a classic vertical Jump impulse with a minimum height and a maximum height as long as Jump input is pushed.
Just try it !
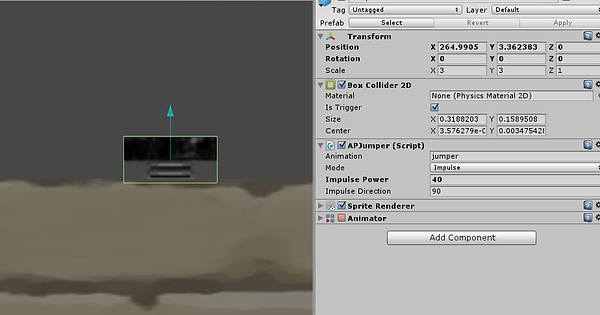
Technical details :
It must have a collider as a trigger for hit detection with the character.
This shape can be any supported 2D shape in Unity.
Just make sure that collision layer is correctly setup (so this layer collides with character's layer).
An animation state is played when player touches the trigger, this animation does not loop and last keyframe is the standby position.
Please use new Animator mechanism otherwise this won't work.
Hitable
A Hitable is a game object for which you want to be notified of some events, for example when hit by a melee attack, touched by a projectile or simply touched by a character.
Create your own script that inherits this script then add it to your game object.
You will have to override some methods for your specific scripting behavior.
Finally add a 2D collider and design your own detection zone.
Notive that character never collides with triggers and then they never receive the OnCharacterTouch events.
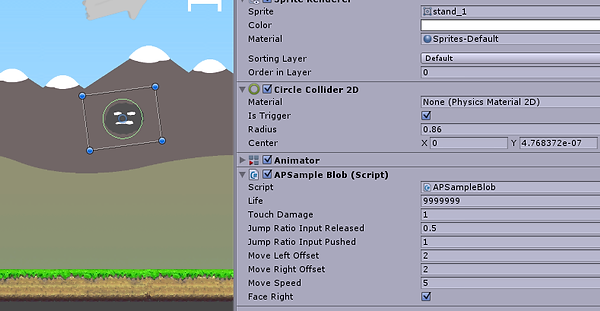
public class APHitable : MonoBehaviour
{
// called when we have been hit by a melee attack
// - launcher : character controller launching the attack
// - hitZone : hitzone detecting the hit
// - return false if you want engine to ignore the hit
virtual public bool OnMeleeAttackHit(APCharacterController launcher, APHitZone hitZone) { return false; }
// called when we have been hit by a bullet
// - launcher : character controller launching the bullet
// - bullet : reference to bullet touching us
// - return false if you want engine to ignore the hit, true if you want bullet to be destroyed
virtual public bool OnBulletHit(APCharacterController launcher, APBullet bullet) { return false; }
// called when character is touching us with a ray
// - motor : character controller touching the hitable
// - rayType : type of ray detecting the hit
// - hit : hit info
// - penetration : penetration distance (from player box surface to hit point, can be negative)
// - return false if contact should be ignored by engine, true otherwise
virtual public bool OnCharacterTouch(APCharacterController launcher, APCharacterMotor.RayType rayType, RaycastHit2D hit, float penetration) { return true; }
}
Please check the script APSampleBlob for more information about scripting your own NPC.
In this sample, we handle the case where the player is jumping on us or touching us.
We also manage a kind of life system for our player and npc.
Check the Melee Attack demo level for more informations.
Samples
A game can be very specific and the package could not cover every aspects of a gameplay.
That's why we try to stay as generic as possible to allow you scripting any kind of behavior.
Some samples scripts are provided to help you in creating your own scripts and behaviors.
Notice that some of theses samples scripts may be interdependent.
All these scripts are well documented and should be easy to understand.
Look at the folder Samples and check the prefabs using this, this will help you a lot creating your own script.
Here is a non exhaustive list of behaviors created by these scripts :
-
Player life system with GUI
-
Restart level when falling in void
-
Falling platform
-
Moving platform when landing on it
-
Basic npc move / hit
-
Breakable crate
-
Collectable (life, ammo...)
-
Vertical down jump
-
Audio events
-
And more...
This is a good place to starts scripting from.
Ask for support if you don't understand something, new samples could be added to the package!